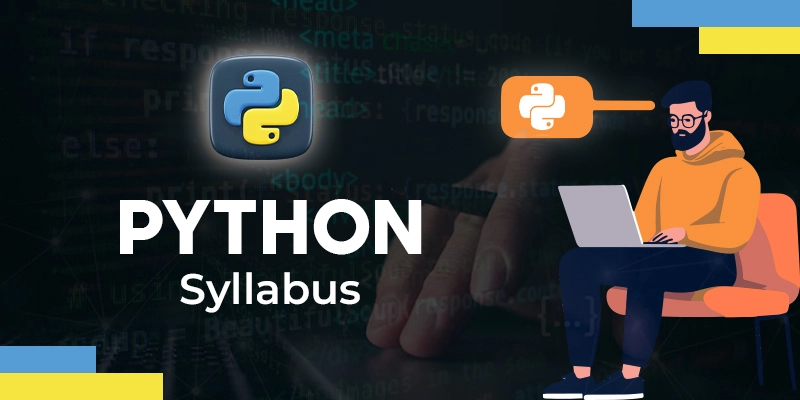
Python is one of the most common programming languages being used today. Our Python Course offers a complete knowledge required to be a successful Python programmer. That being said, lets look into the Python Course Syllabus.
Python Programming
Introduction to Python
We will start with an introduction to the Python programming language. You will learn some of the special features of Python that set it apart from other languages. We also cover important differences between Python 2 and Python 3: enhanced syntax, improved Unicode support, and refreshed standard library in Python 3. You will be learning how to install and configure a Python development environment.
- What is Python and the history of Python
- Unique features of Python
- Python-2 and Python-3 differences
- Installing Python
- Setup Python Development Environment
Important Programming Basics in Python
Next up in the Python Programming Syllabus, we are going to see the basics of programming in Python. We shall take a look at the keywords in Python, indentation, and finally, comments for writing clean code in this language. You will get familiar with primary data types, variables, and operators. The operators include arithmetic and logical operators. Lastly, you will know how to manipulate strings and get user input all the way through to writing your very first Python program.
- Python Keywords and Indentation
- Comments
- Python Basic Data Types
- Python Variables
- Operators in Python
- Strings in Python
- Getting User Input
- First Python Program
If you want to join the ideal Python Course in Chennai, join FITA Academy.
Loops and Control Statements
In this section, we will be looking into the various types of loops and control statements, such as if, nested if, for, while etc.
Control Structures
- Simple if
- if-else
- nested if
- If-elif-else
Loops
- For loop
- while loop
Loops and control statements are a significant part of Python Interview Questions and Answers asked by employers. Its essential to be knowledgeable on the subject.
Break & Continue Statements
In this section, we will be knowing what break and continue statements are and their applications.
Functions, Modules & Packages in Python
In this section, youโll learn to define and call user-defined functions, understand function parameters and scope, and use global vs local variables. Youโll explore lambda and anonymous functions, create and import modules, and use the __name__ and __main__ keywords. Additionally, youโll learn to create packages and utilize both the standard library and external libraries.
- Python user-defined functions
- Defining and calling functions.
- Function parameters
- Function scope and global vs local variables
- Lambda functions
- Anonymous functions
- Creating and using modules
- Importing modules and namespaces
- The __name__ and __main__ keywords
- Creating and using packages
- Using the standard library and external libraries.
Data Structures in Python
In this section, youโll learn about lists in Python, including their use as stacks and queues, and explore tuples. Youโll understand the del statement, iterators, and the use of generators, comprehensions, and lambda expressions. Youโll also learn about ranges, dictionaries and their advanced features, as well as sets with practical examples, providing you with a solid grasp of these essential Python data structures.
- Lists in Python
- Lists as Stacks
- Lists as Queues
- Tuples in Python
- Understanding Del statement
- Understanding Iterators
- Generators, Comprehensions and Lambda
- Expressions
- Understanding and using Ranges
- Python Dictionaries
- More on Dictionaries
- Sets
- Python Sets Examples
Exception Handling in Python
Here, youโll learn about raising and handling exceptions in Python, including creating custom exceptions. Youโll understand how to use try, except, and finally blocks to manage errors and ensure your programs run smoothly, covering essential techniques for robust error handling.
- Raising Exceptions
- Handling Exceptions
- Creating custom Exceptions
- Using try
- Using except
- Using finally
If you are looking for the best place to join the Python Course in Bangalore, look no further than FITA Academy.
Multithreading in Python
In this section of the Python Syllabus, you will learn how to create threads, ensure proper synchronization, and execute concurrency correctly. You will be investigating how using thread pools provides efficient handling of system resources for dealing with tasks and several of the Multiprocessing modules to harness a state-of-the-art many-CPU core machine which improves the performance and responsiveness of a program.
- Creating Threads
- Thread synchronization
- Thread pools
- Multiprocessing Module
File Handling (I/O) in Python
In this module, youโll grasp the essentials of file operations in Python. Learn to read and write text files efficiently. Explore appending data to files and tackle a stimulating challenge. Additionally, you will learn the intricacies of writing binary files, both manually and with Pythonโs Pickle module.
- Reading and writing text files
- Writing Text Files
- Appending to Files and Challenge
- Writing Binary Files Manually
- Using Pickle to Write Binary Files
Collections in Python
Here, youโll grasp the fundamental concepts of collections, namely lists, tuples, sets, and dictionaries. Discover how lists offer ordered collections, tuples provide immutable sequences, sets ensure uniqueness, and dictionaries facilitate key-value mappings.
- Understanding the Basics of Collections
You can learn more about collections by referring to Python Books.
Object Oriented Programming in Python
In this section of the Python Programming Course Syllabus, learn about Object-Oriented Programming in Python, from class definition to inheritance and polymorphism. Explore attribute access and efficient memory management through object destruction. Master these concepts for crafting robust and maintainable Python applications effortlessly.
- Understanding OOPS in Python
- Defining and Using Classes
- Defining and Using Objects
- Mastering Encapsulation in Python
- Mastering Inheritance in Python
- Mastering Polymorphism in Python
- Accessing attributes
- Built-In Class Attributes
- Destroying Objects
Refer to the Python Tutorial to know more about Object Oriented Programming in Python.
Python Regular Expressions
Next up, youโll learn about regular expressions and powerful tools for pattern matching in strings. Weโll explore functions like match and search, understanding the difference between matching and searching. Additionally, youโll discover how to perform search and replace operations efficiently. Weโll delve into extended regular expressions and the utilization of wildcards for versatile pattern matching.
- What are regular expressions?
- The match Function
- The search Function
- Matching vs searching
- Search and Replace
- Extended Regular Expressions
- Wildcard
Database Connectivity in Python
In this section, youโll grasp relational databases and SQLโs significance. Youโll create databases, tables, and insert data, all while honing your querying skills. Additionally, youโll become adept at error handling, ensuring smooth database operations.
- Understanding of relational databases
- Understanding the role of SQL
- Creating and connecting to databases using Oracle or MySQL.
- Understanding the concept of tables
- Understanding fields and primary keys
- Creating tables
- Inserting data into tables
- Querying data using SQL
- Delete records from tables
- Error handling
Master Python with FITA Academyโs immersive Python Course in Pondicherry.
Network Programming
Now you will learn about sockets, unraveling their significance in networking. Explore the dynamics between clients and servers, unraveling their symbiotic relationship in data exchange. Dive into the realm of HTTP requests, mastering the art of handling them to craft seamless web experiences.
- Introduction to Sockets
- Understanding Clients
- Understanding Server
- Handling http requests
Capstone Projects
In this section of the course, we will be looking at a couple of capstone projects. Initially, youโll delve into web scraping, a process of extracting data from websites using Python scripts. This project entails analyzing website structures and employing Python libraries for data extraction. Subsequently, youโll tackle Excel automation, utilizing the win32com module to automate Excel tasks such as data modification and file saving.
- Web Scraping using Python
- Excel Automation using Python
Having these capstone projects as part of your resume will boost your chances of getting a high Python Developer Salary for Freshers.
Django Framework
HTML & CSS Refresher
In this section of the Basic Python Syllabus, youโll learn the essential elements of an HTML document, including understanding and using HTML tags effectively. Weโll explore various HTML editors, create simple HTML documents, and add attributes to tags. Youโll master handling texts, applying HTML styles, and creating tables. Additionally, youโll learn to handle images, layouts, and forms, enabling you to build well-structured and visually appealing web pages.
- HTML
- Elements of a HTML Document
- Understanding HTML Tags
- HTML Editors
- Creating a Simple HTML Document
- Adding Attributes to Tags
- Handling Texts in HTML
- HTML Styles
- HTML Tables
- Handling Images
- Handling Layouts
- HTML Forms
Introducing HTML 5
- HTML Vs HTML 5
- New Elements in HTML 5
- New Form Elements in HTML 5
- Handling Audio & Video
- Handling Graphics
- Handling Events
- HTML 5 APIs
- Web Workers
Introducing CSS and CSS 3
This section of the Python Programming Syllabus at at FITA Academy gets you started with the basics on CSS and CSS3: just what a style sheet is and how it works, cascading. Youโll get to know the difference between inline and external CSS, how to work with selectors, and the use of IDs and classes. Weโll delve into layout techniques, covering absolute, relative, and fixed positioning, and advance your skills with more complex CSS features.
- What is a Style Sheet
- Cascading Effect of a Style Sheet
- Inline and External CSS
- CSS Selectors โ IDs and Classes
- Understanding Layouts
- Absolute, Relative and Fixed Positioning
- Advanced CSS
Introduction of Django Framework
Following CSS, we will be looking into the Django framework, learning about its robust models layer, the installation process, and how to configure the settings module. Youโll also explore handling requests and responses, running the development server, and getting an introduction to the powerful Django admin site.
- Models layer
- Django installation
- Settings module
- Requests and responses
- Running development server
- Django admin site introduction
Models Layer
In this section of the Python Basic Syllabus, youโll learn to install Django, configure the settings module, and handle requests and responses. Youโll explore running the development server, using the Django admin site, and working with models, field types, and queries. Additionally, youโll cover accessing related objects, performing migrations, and managing database transactions, equipping you with essential skills to build robust web applications.
- Django installation
- Settings module
- Requests and responses
- Running development server
- Django admin site introduction
- Model introduction
- Field types and customization
- Queries
- Accessing related objects
- Django migrations
- Database transactions
View Layer
In this module of the Python Developer Syllabus, youโll learn about the view layer in Django, including how to define and use view functions for handling HTTP requests. Youโll explore URL configurations (URLConfs) and understand the use of shortcuts and decorators to simplify common tasks. Additionally, youโll dive into request and response objects, manage file uploads, and work with class-based views and mixins to create reusable and organized code.
- View functions
- Handling HTTP Requests
- URLConfs
- Shortcuts and decorators
- Request and response objects
- File upload
- Class based views
- Mixins
Template Layer
In this Python Course module, you will learn about Djangoโs template layer, how to work with forms, and the template language itself. It covers built-in tags and filters as well as some humanization features of this template language. You will also learn how to roll out your own tags and filters. Mastering CSRF tokens and their place in securing web applications is another key aspect.
- Forms
- Overview of template language
- Built-in tags and filters
- Humanization
- Custom tags and filters
- Csrf token
Forms
In this section of the Python Syllabus for Beginners, youโll learn about the essentials of Djangoโs template layer, including creating and managing forms using the Forms API, validating form data, and utilizing built-in fields and widgets. Additionally, youโll explore model forms and form sets, which streamline form creation and management based on Django models.
- Introduction
- Forms API
- Validating forms
- Built-in fields, built-in widgets
- Model form
- Form sets
Internationalization and Localization
This chapter will cover internationalization and localization. You will see ways of setting both user interface elements and form input based on languages and regions. Besides, you will also learn various best practices in dealing with TimeZones that will go a long way in providing a seamless user experience across global audiences.
- Internationalization
- Localization
- Localizing UI and form inputs
- Time zones
Important Web Application Tools
In this section of the Advanced Python Syllabus, youโll learn important web application tools, including Djangoโs built-in authentication, password management, and how to customize authentication. Youโll also explore caching, logging, pagination, session handling, and static file management. Additionally, youโll be introduced to the Bootstrap framework, enhancing your ability to create responsive and visually appealing web applications.
- Authentication
- Django built-in
- authentications
- Password management
- Customizing authentication
- Caching
- Logging
- Pagination
- Sessions
- Static file management.
- Introduction to the bootstrap framework
Capstone Project
In this part of the Python Syllabus, we will be dealing with the Capstone project, which involves creating a website using Python and Django. A web application will also be created where users can read and write blog posts, administer user accounts, leave comments for other users, and many other functions.