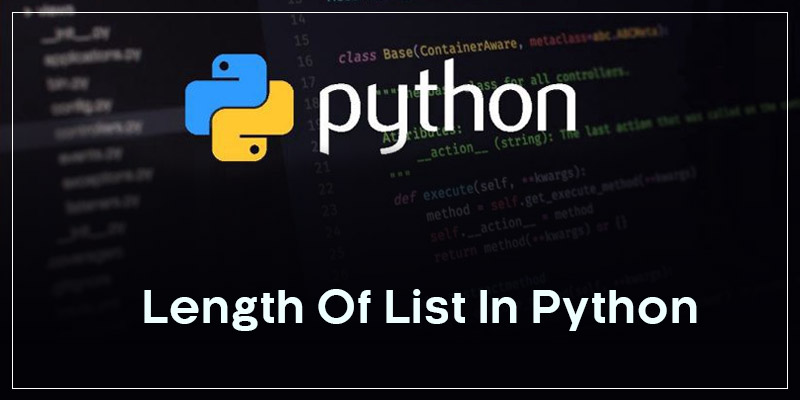
You will often need to group several elements in your program in order to process them as a single object.For this you will need to use different methods in collections.One of the most useful methods in collections module, in Python is a list.
Table Of Content
Lists In Python
A list contains is a collection of multiple elements or items or arbitrary objects (in the programming sense).They are like arrays in the other languages but more versatile and flexible.
Eg:
my_list=[1,‘fita’,’training’,[‘chennai’,’bangalore’],3]
Characteristics Of Lists
Methods In Lists
{Note that the indexing starts at 0}
There are many more useful methods in lists and you can grab insights of them, dynamically slicing lists and their powerful applications including real-time projects with live assistance, in Python Online Training by FITA.
Program 1: Finding the length of list using len method
len is a built-in method in python which returns the size of the object passed to it, irrespective of the index of last element.When a string is passed as an argument it returns the number of characters in the string.It is often used technique in programs these days.
my_list=[]
my_list.append(‘hello’,’readers’)
print(‘length of our list: ‘,len(my_list))
Output:
Length of our list: 3
Program 2: Finding the length of list with a custom method.
my_list=[0,1,1,2,3,5]
counter=0
for _ in my_list:
counter+=1
print(‘size of list with my method: ‘,counter)
Output:
size of list with my method: 6
Program 3: Find the length of the nested list
Nested lists have lists as an element in them. You can consider a nested list as a matrix..We can find the length of such lists, The length of the list can be using len method and the length of a sub list can be found by passing the sub list as argument to len method.
my_list=[[‘list 1’,‘element 1’,’element 2’],[‘list 2’, ‘element 1’, ‘element 2’]]
print(‘length of the list: ’,len(my_list))
print(‘length of the first sub list: ’,len(my_list[0]))
Output:
length of the list: 2
length of the first sub list: 3
The other ways to find the length of the list includes using length_hint() and __len__ method. Now you can also write your own unique program to find the length of the nested list using for or while loops.
Time Complexity Of len function
len() function actually calls the __len__ method internally to return the size of the object and every object (list, tuple, set, dictionary, set, array.array) has its own __len__ method, so all it does is return self.length.
So the time complexity of the len() method is O(1) (in big-O notation) or constant time.
To get in-depth knowledge of Python along with its various applications and real-time projects, you can enroll in Python Training in Chennai or Python Training in Bangalore by FITA at an affordable price, which includes certification, support and career guidance assistance.