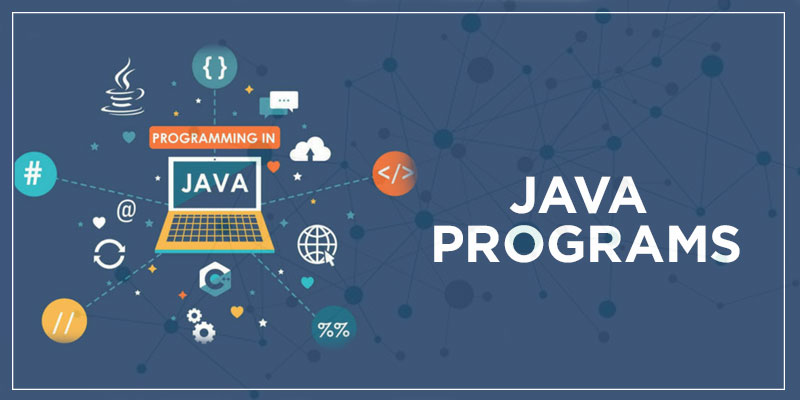
Here are the 13 java programs for you to practice and hone your programming skills with java.
Java Programs on numbers
Programs On Strings In Java
Programs On Arrays In Java
Recursion Programs in Java
Searching/Sorting Program In Java
Java Programs on numbers
import java.util.Scanner;
public class Fibonacci{
public static void main(String args[]) {
System.out.println("How many Fibonacci terms do you want? ");
// creating a scanner object
Scanner scn = new Scanner(System.in);
int counter = scn.nextInt(); // taking the input with scanner
int a = 0;
int b = 1;
int c, k;
System.out.print(a + " " + b); // fib value for 0 and 1
for (k = 2; k < counter; ++k) // looping starts from 2
{
c = a + b;
System.out.print(" " + c);
a = b;
b = c;
}
scn.close();
}
}
Output for the above program
How many Fibonacci terms do you want? 7
0 1 1 2 3 5 8
import java.util.Scanner;
public class Main {
public static void main(String args[]) {
System.out.println("Find the factorial of: ");
// creating a scanner object
Scanner scn = new Scanner(System.in);
int number = scn.nextInt(); // taking the input with scanner
int k, fact = 1; // first factorial value
for (k = 1; k <= number; k++) {
fact = fact * k;
}
System.out.println("Factorial of " + number + " is: " + fact);
scn.close();
}
}
Output for the above program
Find the factorial of:
6
Factorial of 6 is: 720
import java.util.Scanner;
public class Leap{
public static void main(String[] args) {
System.out.println("Check leap year for: ");
Scanner scn = new Scanner(System.in); // scanner object
int yr = scn.nextInt(); // taking the input with scanner
boolean leap_yr = false;
if (yr % 4 == 0) {
if (yr % 100 == 0) {
// if yr is divisible by 400 it is a leap year
if (yr % 400 == 0)
leap_yr = true;
else
leap_yr = false;
} else
leap_yr = true;
} else
leap_yr = false;
if (leap_yr)
System.out.println(yr + " is a leap year.");
else
System.out.println(yr + " is not a leap year.");
scn.close();
}
}
Output 1 for the above program
Check leap year for:
1990
1990 is not a leap yr.
Output 2 for the above program
Check leap year for:
2020
2020 is a leap year.
Programs On Strings In Java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
System.out.println("Reversed string for: ");
Scanner scn = new Scanner(System.in); // scanner object
String str = scn.nextLine(); // taking the input with scanner
// For storing the reverse of the given string
String reversedStr = "";
// Iterate string from last and add each character to reversedStr
for (int s = str.length() - 1; s >= 0; s--) {
reversedStr = reversedStr + str.charAt(s);
}
System.out.println("Original string: " + str);
//reverse of the given string
System.out.println("Reversed string: " + reversedStr);
scn.close();
}
}
Output for the above program
Reversed string for: year
Original string: year
Reversed string: raey
import java.util.Arrays;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// creating an object of Scanner class for input
Scanner scn = new Scanner(System.in);
// taking input from users with scn
System.out.print("First String: ");
String str_1 = scn.nextLine();
System.out.print("Second String: ");
String str_2 = scn.nextLine();
if(str_1.length() == str_2.length()) {
// converting strings to arrays
char[] arr_1 = str_1.toCharArray();
char[] arr_2 = str_2.toCharArray();
// sorting the arrays
Arrays.sort(arr_1);
Arrays.sort(arr_2);
boolean ang = Arrays.equals(arr_1, arr_2);
if(ang) {
System.out.println("The strings are valid anagram.");
}
else {
System.out.println("The strings are not valid anagram.");
}
}
else{
System.out.println("The strings are not valid anagrams");
}
scn.close();
}
}
Output for the above program
First String: reader
Second String: read
The strings are not valid anagrams
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
int vowel = 0;
int consonant = 0;
// creating an object of Scanner for input
Scanner scn = new Scanner(System.in);
// taking input from users with scn
System.out.print("Your String: ");
String strng = scn.nextLine();
input = input.toLowerCase();
for (int s = 0; s < strng.length(); s++)
{
if (strng.charAt(s) == 'a' || strng.charAt(s) == 'e' || strng.charAt(s) == 'i' || strng.charAt(s) == 'o'
|| strng.charAt(s) == 'u') {
// Increments the vowel counter
vowel++;
} else if (strng.charAt(s) >= 'a' && strng.charAt(s) <= 'z') {
consonant++;
}
}
System.out.println("vowels: " + vowel);
System.out.println("consonants: " + consonant);
scn.close();
}
}
Output for the above program
Your String: the simplest string ever
Number of vowels: 6
Number of consonants: 15
Check out this Complete Java Online Course by FITA. FITA provides a complete Java course including core java and advanced java J2EE, and SOA training, where you will be building real time applications using Servlets, Hibernate Framework, and Spring with Aspect Oriented Programming (AOP) architecture, Struts through JDBC bundled with, placement support, and certification at an affordable price with an active placement cell, to make you an industry required certified java developer.
Programs On Arrays In Java
public class Duplicate{
public static void main(String[] args) {
int[] array = new int[] { 1, 1, 2, 3, 5, 2, 3, 5, 7 };
System.out.println("Founded these duplicate elements: ");
for (int r = 0; r < array.length; r++) {
for (int c = r + 1; c < array.length; c++) {
if (array[r] == array[c])
System.out.println(array[c]);
}
}
}
}
Output for the above program
Founded these duplicate elements:
1
2
3
5
public class Sort {
public static void main(String[] args) {
// Initialize the original array
int[] array = new int[] { 4,6,7,1,2,4 };
int tmp = 0;
int len = array.length
// Sort the array in ascending order
for (int h = 0; h < len; h++) {
for (int c = h + 1; c < len; c++) {
if (array[h] > array[c]) {
tmp = array[k];
array[h] = array[c];
array[c] = tmp;
}
}
}
System.out.println();
// Elements of array after sort
System.out.println("Array after sorting: ");
for (int h = 0; h < len; h++) {
System.out.print(array[h] + " ");
}
}
}
Output for the above program
Sorted elements:
1 2 4 4 6 7
public class Main {
public static void main(String[] args) {
// Initialize array
int[] arr = new int[] { 1, 1, 2, 3, 5 };
len = arr.length
System.out.println("reversed array : ");
// Looping through array from last
for (int h = len - 1; h >= 0; h--) {
System.out.print(arr[h] + " ");
}
}
}
Output for the above program
reversed array :
5 3 2 1 1
Recursion Programs in Java
class Palindrome {
static int pal(int num, int tmp) {
// base case
if (num == 0)
return tmp;
tmp = tmp * 10 + num % 10;
return pal(num / 10, tmp);
}
public static void main(String[] args) {
int num = 1221;
int tmp = pal(num, 0);
if (tmp == num)
System.out.println("A palindrome number");
else
System.out.println("Not a palindrome number");
}
}
Output for the above program
A palindrome number
public class ReversedString {
public String reverse(String strng){
if(strng.isEmpty()){
return strng;
} else {
return reverse(strng.substring(1))+strng.charAt(0);
}
}
public static void main(String[] args) {
ReversedString obj = new ReversedString();
String result = obj.reverse("Fita Academy");
System.out.println(result);
}
}
Output for the above program
ymedacA atiF
import java.util.Arrays;
import java.util.Scanner;
class Main {
// method to perform bubble sort
void bubble(int arry[]) {
int len = arry.length;
// for getting elements from array arry
for (int m = 0; m < len - 1; m++) {
// for comparison
for (int n = 0; n < len - m - 1; n++) {
// comparing with the next element
if (arry[n + 1] < arry[n]) {
// performing swap
int temp = arry[n];
arry[n] = arry[n + 1];
arry[n + 1] = temp;
}
}
}
}
// driver method
public static void main(String args[]) {
int[] array = { -2, 4, -6, 0, 9 };
// creating an object of Main
Main bs = new Main();
bs.bubble(array);
System.out.println("Sorted array:");
// for converting int to string
System.out.println(Arrays.toString(array));
}
}
Output for the above program
Sorted array:
[-6, -2, 0, 4, 9]
import java.util.Scanner;
import java.util.Arrays;
class Main {
int binarySearch(int arr[], int element, int lower, int higher) {
while (lower <= higher) {
// get index of middle element
int middle = lower + (higher - lower) / 2;
// if element to be searched is middle
if (arr[middle] == element)
return middle;
// if element is lesser than middle
// search on the left of middle
if (arr[middle] < element)
lower = middle + 1;
// if element is greater than middle
// search on the right of middle
else
higher = middle - 1;
}
return -1;
}
public static void main(String args[]) {
// create an object of the Main class
Main obj = new Main();
int[] arr = { 3, 4, 5, 6, 7, 8, 9 };
int n = arr.length;
// creating a scanner object to take input from a user
Scanner scn = new Scanner(System.in);
System.out.println("Element to be searched for:");
int element = scn.nextInt();
scn.close();
int res = obj.binarySearch(arr, element, 0, n - 1);
if (res == -1)
System.out.println("Element not found in the array");
else
System.out.println("Element found in array at index " + res);
}
}
Output for the above program
Element to be searched for:
5
Element found in array at index 2
These were few practice programs for java. To get in depth knowledge of core Java and advanced java, J2EE and SOA training along with its various applications and real-time projects using Servlets, Spring with Aspect Oriented Programming (AOP) architecture, Hibernate Framework,and Struts through JDBC you can enroll in Certified Java Training in Chennai or Certified Java Course in Bangalore by FITA or a virtual class for this course,at an affordable price, bundled with real time projects, certification, support, and career guidance assistance and an active placement cell, to make you an industry required certified java developer.
FITA’s courses training is delivered by professional experts who have worked in the software development and testing industry for a minimum of 10+ years, and have experience of working with different software frameworks and software testing designs.