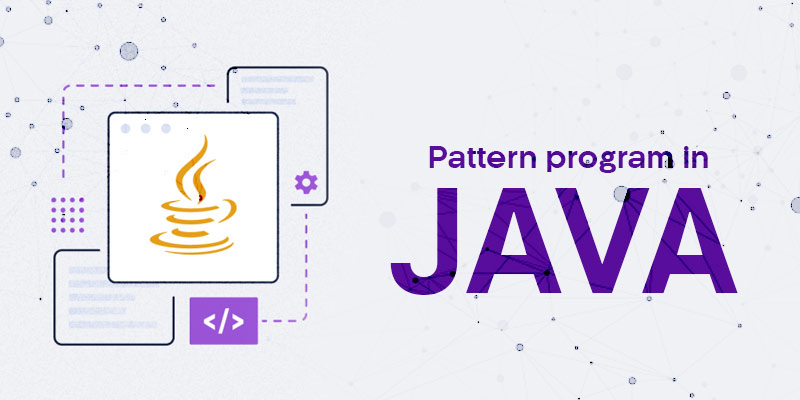
Star Pattern Programs In With Java
import java.util.Scanner;
public class RightHalfPyramid{
public static void main(String[] args) {
System.out.println("Enter the number of rows for pattern: ");
Scanner sc = new Scanner(System.in); // taking the input with scanner
int rows = sc.nextInt();
for(int row = 1; row <= rows; ++row) {
for(int col = 1; col <= row; ++col) {
System.out.print("* ");
}
System.out.println();
sc.close();
}
}
Enter the number of rows for pattern: 5
*
* *
* * *
* * * *
* * * * *
import java.util.Scanner;
public class LeftHalfPyramid
{
public static void main(String[] args)
{
System.out.println("Enter the number of rows for pattern: ");
Scanner sc = new Scanner(System.in); // taking the input with scanner
int rows = sc.nextInt();
for (int row=1; row<=rows; row++)
{
// Printing space in decreasing order
for (int col=rows; col>row; col--)
{
System.out.print(" ");
}
// Printing star in increasing order
for (int star=1; star<=row; star++)
{
System.out.print("*");
}
System.out.println();
}
sc.close();
}
}
Enter the number of rows for pattern: 5
*
**
***
****
*****
import java.util.Scanner;
public class FullPyramid
{
public static void main(String[] args)
{
System.out.println("Enter the number of rows for pattern: ");
Scanner sc = new Scanner(System.in); // taking the input with scanner
int rows = sc.nextInt();
for (int row=1; row<=rows; row++)
{
// Print space in decreasing order
for (int col=rows; col>row; col--)
{
System.out.print(" ");
}
// Print star in increasing order
for (int star=1; star<=row; star++)
{
System.out.print("* ");
}
System.out.println();
}
sc.close();
}
}
Enter the number of rows for pattern: 5
*
* *
* * *
* * * *
* * * * *
import java.util.Scanner;
ย
public class InvertedHalfPyramid {
ย
public static void main(String[] args) {
ย
System.out.println("Enter the number of rows for pattern: ");
Scanner sc = new Scanner(System.in); // taking the input with scanner
int rows = sc.nextInt();
ย
for(int row = rows; row >= 1; --row) {
for(int col = 1; col <= row; ++col) {
System.out.print(" * ");
}
System.out.println();
}
sc.close();
}
}
Enter the number of rows for pattern: 5
* * * * *
* * * *
* * *
* *
*
import java.util.Scanner;
public class InvertedHalfPyramid
{
public static void main(String[] args)
{
System.out.println("Enter the number of rows for pattern: ");
Scanner sc = new Scanner(System.in); // taking the input with scanner
int rows = sc.nextInt();
for (int row= rows; row>= 1; row--)
{
for (int col=rows; col>row;col--)
{
System.out.print(" ");
}
for (int count=1;count<=row;count++)
{
System.out.print("*");
}
System.out.println("");
}
sc.close();
}
}
Check out this Complete Online Java Course by FITA. FITA provides a complete Java course including core java and advanced java J2EE, and SOA training, where you will be building real time applications using Servlets, Hibernate Framework, and Spring with Aspect-Oriented Programming (AOP) architecture, Struts through JDBC bundled with, placement support, and certification at an affordable price with an active placement cell, to make you an industry required certified java developer.
Output for the above program
Enter the number of rows for pattern: 5
*****
****
***
**
*
import java.util.Scanner;
public class InvertedFullPyramid
{
public static void main(String[] args)
{
System.out.println("Enter the number of rows for pattern: ");
Scanner sc = new Scanner(System.in); // taking the input with scanner
int rows = sc.nextInt();
for (int row= rows; row>= 1; row--)
{
for (int col=rows; col>row;col--)
{
System.out.print(" ");
}
for (int count=1;count<=row;count++)
{
System.out.print(" *");
}
System.out.println("");
}
sc.close();
}
}
Enter the number of rows for pattern: 5
* * * * *
* * * *
* * *
* *
*
import java.util.Scanner;
public class Diamond
{
public static void main(String[] args)
{
// Scanner object
Scanner scanner = new Scanner(System.in);
// taking the number of rows with the scanner
System.out.println("Enter the number of rows for pattern: ");
int rows = scanner.nextInt();
for (int row=1; row<=rows; row++)
{
// Print space in decreasing order
for (int col=rows; col>row; col--)
{
System.out.print(" ");
}
// Print star in increasing order
for (int count=1; count<=(row * 2) -1; count++)
{
System.out.print("*");
}
System.out.println();
}
for (int row=rows-1; row>=1; row--)
{
// Printing space in increasing order
for (int col=rows-1; col>=row; col--)
{
System.out.print(" ");
}
// Printing stars in decreasing order
for (int count=1; count<=(row * 2) -1; count++)
{
System.out.print("*");
}
System.out.println();
}
scanner.close();
}
}
Enter the number of rows for pattern: 5
*
***
*****
*******
*********
*******
*****
***
*
import java.util.Scanner;
public class SandGlass {
public static void main(String[] args) {
// scanner object
Scanner sc = new Scanner(System.in);
System.out.println("Enter the number of rows for pattern: ");
// taking the number of rows with scanner
int rows = sc.nextInt();
for (int row = 0; row <= rows - 1; row++) {
for (int col = 0; col < row; col++) {
System.out.print(" ");
}
for (int count = row; count <= rows - 1; count++) {
System.out.print("* ");
}
System.out.println("");
}
for (int row = rows - 1; row >= 0; row--) {
for (int col = 0; col < row; col++) {
System.out.print(" ");
}
for (int count = row; count <= rows - 1; count++) {
System.out.print("* ");
}
System.out.println("");
}
sc.close();
}
}
Enter the number of rows for pattern: 5
* * * * *
* * * *
* * *
* *
*
*
* *
* * *
* * * *
* * * * *
Check out this Complete Online Java Training by FITA. FITA provides a complete core and advanced Java course where you will be building real-time applications using J2EE, Servlets, Hibernate Framework, and Spring with Aspect-Oriented Programming (AOP) architecture, Struts through JDBC bundled with, placement support, and certification at an affordable price with an active placement cell, to make you an industry-certified java developer.
After the star pattern programs, we will now see the numeric pattern programs in java
Numeric Pattern Programs With Java
import java.util.Scanner;
public class Main
{
public static void main(String[] args)
{
// scanner object
Scanner sc = new Scanner(System.in);
System.out.println("Enter the number of rows for pattern: ");
int n = sc.nextInt(); // taking input with scanner sc
int row, col,number;
for(row=0; row<n; row++) // outer loop for rows
{
number=1;
for(col=0; col<=row; col++) // inner loop for rows
{
// adding a space after number
System.out.print(number+ " ");
//incrementing value of number
number++;
}
// printing an empty line
System.out.println();
}
}
}
Enter the number of rows for pattern:
5
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
import java.io.*;
import java.util.Scanner;
public class NumberPattern {
public static void printNums(int num) {
// initialising starting number
int row, col, number = 1;
for (row = 0; row < num; row++) {
for (col = 0; col <= row; col++) {
// // adding a space after number
System.out.print(number + " ");
// incrementing number at every column
number = number + 1;
}
// ending line after each row
System.out.println();
}
}
public static void main(String args[]) {
// scanner object
Scanner sc = new Scanner(System.in);
System.out.println("Enter the number of rows for pattern: ");
int num = sc.nextInt(); // taking input with scanner sc
printNums(num);
sc.close();
}
}
Enter the number of rows for pattern: 6
1
2 3
4 5 6
7 8 9 10
11 12 13 14 15
16 17 18 19 20 21
import java.io.*;
import java.util.Scanner;
public class PascalPattern {
public static void main(String[] args) {
//scanner object
Scanner sc = new Scanner(System.in);
System.out.println("Enter the number of rows pattern: ");
// taking input with scanner
int rows = sc.nextInt();
int number = 1;
for(int row = 0; row < rows; row++) {
for(int space = 1; space < rows - row; ++space) {
System.out.print(" ");
}
for(int col = 0; col <= row; col++) {
if (col == 0 || row == 0)
number = 1;
else
number = number * (row - col + 1) / col;
System.out.printf("%4d", number);
}
// printing new line after iterations
System.out.println();
}
}
}
Enter the number of rows for pattern: 6
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
1 5 10 10 5 1
import java.util.Scanner;
public class RepeatedPattern
{
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in); //Taking rows value from the user
System.out.println("Enter the number of rows for pattern: ");
int rows = sc.nextInt();
for (int i = 1; i <= rows; i++)
{
for (int j = 1; j <= i; j++)
{
System.out.print(i+" ");
}
System.out.println();
}
sc.close();
}
}
Enter the number of rows for pattern: 6
1
2 2
3 3 3
4 4 4 4
5 5 5 5 5
6 6 6 6 6 6
import java.io.*;
import java.util.Scanner;
public class HalfPyramid {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in); // Scanner Object
System.out.println("Enter the number of rows for pattern: ");
// Taking rows value from the user with scanner
int rows = sc.nextInt();
for(int row = rows; row >= 1; --row) {
for(int col = 1; col <= row; ++col) {
System.out.print(col + " ");
}
System.out.println();
}
sc.close();
}
}
Enter the number of rows for pattern: 6
1 2 3 4 5 6
1 2 3 4 5
1 2 3 4
1 2 3
1 2
1
import java.io.*;
import java.util.Scanner;
public class MainClass
{
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in); // Scanner Object
System.out.println("Enter the number of rows for pattern: ");
int rows = sc.nextInt(); // Taking rows value from the user with scanner
for (int row = 1; row <= rows; row++)
{
for (int col = 1; col <= row; col++)
{
System.out.print(col+" ");
}
for (int col = row-1; col >= 1; col--)
{
System.out.print(col+" ");
}
System.out.println();
}
sc.close();
}
}
Enter the number of rows for pattern: 6
1
1 2 1
1 2 3 2 1
1 2 3 4 3 2 1
1 2 3 4 5 4 3 2 1
1 2 3 4 5 6 5 4 3 2 1
Character Pattern Programs With Java
import java.io.*;
import java.util.Scanner;
public class RightAlphaTri
{
public static void main(String[] args)
{
int alphabet = 65; //ASCII value of A is 65
Scanner sc = new Scanner(System.in); // Scanner Object
System.out.println("Enter the number of rows for pattern: ");
// Taking rows value from the user with a scanner
int rows = sc.nextInt();
for (int row = 0; row <= rows; row++)
{
for (int col = 0; col <= row; col++)
{
System.out.print((char) (alphabet + col) + " ");
}
System.out.println();
}
sc.close();
}
}
Enter the number of rows for pattern: 6
A
A B
A B C
A B C D
A B C D E
A B C D E F
A B C D E F G
import java.io.*;
import java.util.Scanner;
public class Edureka
{
public static void main(String[] args)
{
int alphabet = 65;
Scanner sc = new Scanner(System.in); // Scanner Object
System.out.println("Enter the number of rows for pattern: ");
// Taking rows value from the user with a scanner
int rows = sc.nextInt();
for (int row = 0; row<= rows; row++)
{
for (int col = 0; col <= row; col++)
{
System.out.print((char) alphabet + " ");
}
alphabet++;
System.out.println();
}
sc.close();
}
}
Enter the number of rows for pattern: 6
A
B B
C C C
D D D D
E E E E E
F F F F F F
import java.io.*;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in); // Scanner Object
System.out.println("Enter the number of rows for pattern: ");
// Taking rows value from the user with scanner
int rows = sc.nextInt();
int alphabet = 65;
for (int row = 0; row <= rows; row++) {
for (int col = 8; col > row; col--) {
System.out.print(" ");
}
for (int count = 0; count <= row; count++) {
System.out.print((char) (alphabet + count) + " ");
}
System.out.println();
}
sc.close();
}
}
Enter the number of rows for pattern: 6
A
A B
A B C
A B C D
A B C D E
A B C D E F
A B C D E F G
This was all printing patterns using java. To get in-depth knowledge of core Java and advanced java, J2EE SOA training along with its various applications and real-time projects using Servlets, Spring with Aspect-Oriented Programming (AOP) architecture, Hibernate Framework, and Struts through JDBC you can enroll in Certified Java Training in Chennai or Certified Java Training in Bangalore by FITA or a virtual class for this course, at an affordable price, bundled with real-time projects, certification, support, and career guidance assistance and an active placement cell, to make you an industry required certified java developer.
FITAโs courses training is delivered by professional experts who have worked in the software development and testing industry for a minimum of 10+ years, and have experience of working with different software frameworks and software testing designs.