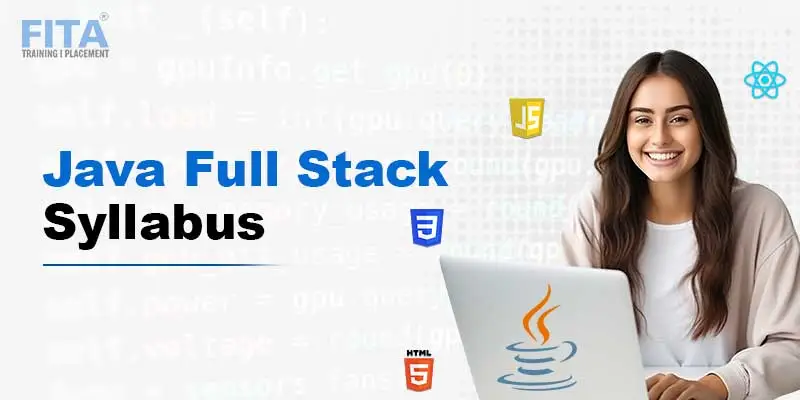
A comprehensive Java Full Stack Developer Course Syllabus encompasses a broad spectrum of subjects, equipping learners with client- and server-side development expertise. The course focuses on leveraging Java for robust back-end systems and Angular for creating interactive, responsive front-end applications.
Core Java Programming
This Java Full Stack Developer Course Syllabus begins with a foundation in Java and object-oriented programming concepts. Youโll explore the structure of Java programs, including various data types and operators. The course covers essential elements like variable usage, method creation, and object instantiation, preparing you for more complex topics in software development.
Introduction to Java
In the Introduction to Java module, you will receive an overview of Object-Oriented Programming basics, where you learn about classes, objects, inheritance, and polymorphism.
- Object Oriented Programming Fundamentals
- Structure of a Java Program
Data Types
In this module, you will learn various data types relevant to programming in detail. In this Java Full Stack Developer Syllabus, you will learn about primitive data types that efficiently store simple values, such as integers and booleans.
- Primitive data types
- Reference Data Types
- Keywords, Identifiers, Expressions
Operators
Master the essential operators at the root of programming. You will closely examine arithmetic operators for simple mathematical operations, assignment operators to operate with variables, and logical operators to have extracted for decisions.
- Arithmetic Operators
- Assignment Operators
- Logical Operators
- Relational Operators
- Bitwise Operators
Variables
You will learn about variables, how they are declared and defined, what types of variables exist, how to use them, and when.
- Declaration, Definition, Types
Methods
You will dive deep into the mechanics, their syntax, and various types. You will learn how to define and call a method, how instance and class methods differ, and how method overloading works in this Full Stack Java Syllabus.
- Syntax, Types
Object
In this module, we will look at the creation of objects and reference variables. You will learn how instances of objects are created in memory and how the reference variables point to the created objects.
- Object Creation, Reference, Reference Variables
Constructors
Understand constructors and their use in object initializations. Learn about pass by reference and pass by value to see how data is treated inside functions. Get a handle on access specifiers and access levels for visibility and access control.
- Pass by Value and Pass by Reference
- Access Specifiers
- Access Levels
- Decision Making and Control Structures
Strings
In this section of the Java Full Stack Course Syllabus, we delve into strings; we differentiate between String, StringBuffer, and StringBuilder in Java.
- String, String Buffer, String Builder
Java Beans
Java Beans are reusable building blocks for modular and maintainable Java applications. Learn to create, configure, and use them for enterprise app development.
Arrays
In this module, you will learn how to declare and define arrays and about primitive and object arrays.
- Declaring and defining arrays
- Primitive Arrays.
- Object Arrays
Inheritance
In this module, you will learn inheritance and composition, understand โis-aโ and โhas-aโ relationships, and use extends and implements keywords.
- Is-A Relationship
- Has-A Relationship
- Inheritance using extends keyword
- Inheritance using implements Keyword
Abstraction
In this module of the Java Full Stack Course Syllabus, you will learn how abstraction works by using abstract classes and interfaces.
- Abstraction using abstract classes
- Abstraction using interfaces
Encapsulation
You will learn how to apply encapsulation correctly to achieve maximum code reliability and modularity in front-end and back-end development scenarios.
Interfaces
In this module, you will clearly differentiate between interfaces and classes in terms of how each defines behavior and structure in object-oriented programming.
- Interfaces Vs Classes
- Nested Interfaces
- Interface as a type
Polymorphism
You will learn how to overload methods, where multiple methods in a single class have the same name but differ in their parameters, and constructor overloading, where you can have more than one constructor in your class but with a different parameter list.
- Overloading
- Constructor overloading
- Overloading between classes
- Overriding
Exception Handling
This module of the Java Full Stack Developer Syllabus will help you understand exceptions and their different types in the hierarchy of exceptions.
- Exception
- Categories of Exception, Exception hierarchy
- Throw and throws keywords
- Try catch and finally keywords
Collection Framework
This module delves into collection frameworks and familiarises you with the crucial core interfaces and classes comprising the Java backbone of its power in handling data.
- Core Interfaces
- Core Classes
- Iterator
- Comparable & Comparator
Generics
You will learn how Generics enable type-safe collections and reusable algorithms, thus promoting flexibility and performance in code.
- Autoboxing
- Unboxing
Casting
You will learn about the idea of casting in programming as it relates to primitive and reference types in this Java Full Stack Developer Course Syllabus module.
- Primitive Casting
- Reference Casting
- Upcasting and downcasting
File Handling
In this module, youโll learn file and stream management and their types.
- File Handling in Java
- Files, Streams, Types of Streams
Serialization
In this module, you will look into serialisation, an important concept in storing and transmitting Java objects.
- Thread Life Cycle
- Thread States
- Creating Threads
- Threads Priorities
- Thread Groups
- Synchronization
Master the details of these topics with our Java Full Stack Developer Course in Chennai.
Threads
This module introduces you to Java threading, where youโll explore intricate details about threadsโ life cycles, states, and creation.
- Thread Life Cycle
- Thread States
- Creating Threads
- Threads Priorities
- Thread Groups
- Synchronization
Inner Classes
In this module of the Java Full Stack Syllabus, you will become acquainted with Java inner classes, nested classes, and anonymous classes.
- Nested Classes
- Anonymous Classes
Java Enterprise Edition (JEE)
In this section, you will master Java Enterprise Edition and scale robust, secure enterprise-level applications. Youโll be able to cover the core topics of Servlets, JSP, and EJB, and explore some of the more advanced topics such as JPA, JMS, and Web Services. By the end of this course, students will be able to architect and deploy complex Java applications in a real-world enterprise setup. If you want to get a job with a high paying Full Stack Developer Salary For Freshers, knowledge of JEE is essential.
Overview of J2EE and WWW
This module of the Java Full Stack Syllabus gives an overview of J2EEโJava 2 Platform, Enterprise Editionโa robust platform for building enterprise-level applications and their integration with the World Wide Web.
The HTTP Protocol and Web Application Introduction
You will learn about the basics of HTTP and its generally known, very critical role in communicating over the web.
Environmental Setup
In this module, we will look at how to set up your JEE development environment.
HTML
In this module, you will learn about the basics of the backbone of web development: HTML. You will learn about the new features of HTML5 and master how to structure web pages with elements, tags, and attributes in this Java Full Stack Syllabus.
Servlets
In this module, you will learn about one of the cornerstones of Java Web Development: servlets. We will study the lifecycle of Servlets, their configuration and deployment, and various types of Servlets.
- What is a Servlet?
- Servlet Lifecycle, Configuring a Servlet, Types of Servlet
- Servlet Context
- Servlet Config
- Deployment descriptor
- Session Management
Java Server Pages(JSP)
In this module, you will explore Java Server Pages (JSPs), learn about the JSP lifecycle, and understand how JSPs differ from Servlets.
- JSP Lifecycle
- Servlets vs JSP
- Scriptlets
- Directives
- Declaration
- Sessions
- Mixing Scriptlets and HTML
- Tag Libraries
- Beans and Forms Processing
MVC Architecture
In this module of the Java Full Stack Syllabus,you will learn how MVC, an architectural design pattern in software development, completely covers data, represented by the Model, a display of data in the View, and finally, input handling by the Controller.
JDBC
You will be introduced to MySQL databases and given an overview of JDBC. This will teach you about the different types of JDBC drivers and how JDBC works.
- Database Setup (MySQL)
- Overview
- JDBC Driver Types
- How JDBC Works
- Steps Involved
- JDBC Process details
- Queries
- Prepared Statements
- Callable Statements
Spring and Spring Boot Framework
In this module of the Full Stack Course at FITA Academy, youโll explore Spring and Spring Boot more in-depth. You will learn to write robust, scalable Java applications using Spring. Become proficient at the core ideas: Dependency Injection and Aspect Oriented Programming. Learn about how to design with the MVC architecture. Additionally, you will become familiar with Spring Boot for fast setup and production-ready deployable applications with minimal configuration.
Spring: Introduction
In this module of the Full Stack Java Developer Syllabus, youโll be introduced to the main concepts of the Spring Framework, where youโll learn what Spring is, why itโs used in modern Java development,
- What is Spring
- Advantages of the Spring Framework
- Spring Framework Architecture and Spring Modules
- Java and Spring Configuration
Spring: Core Container
Youโll learn about major concepts like Dependency Injection, Inversion of Control, tight versus loose coupling of object relations, and the life cycle of Spring beans in this module.
- Components of Spring Core
- Object Coupling โ Tight and Loose Coupling
- Dependency Injection (DI)
- Types of DI
- Concepts and Implementation of Inversion of Controls (IoC)
- Spring Bean โ Properties, Scope, Method, Bean Lifecycle
- DI with Bean
- Spring BeanFactory
- Wiring Beans
- AutoWiring Beans
- Understanding the Default AutoWiring
- AutoWiring by Name and AutoWiring by Constructors
- Annotations in Spring
- Dependency Injection using Annotations
- Wiring Bean with Annotations
- Introduction to Spring Expression Language (SPEL)
- SPEL Operators
- Implementing Annotations with SPEL
Spring: Data Access and Integration
This Full Stack Java Syllabus module covers important techniques you will need to work with, such as JDBC, for efficient interaction with databases.
- Data Access with Spring using JDBC
- JDBC Templates
- Data Access Object Patterns (DAO) and Bean
- Querying Database and Binding the Variables
- Handling Database Exceptions
- Executing Update and Delete Statements
- Batch Update data
- Database Transactions
- Transactions Management with Spring
Spring: Web Layer
In the module on Spring Web Layer, you will get to know about the powerful Spring MVC architecture and its different components, and how to set up applications effectively.
- ISpring Model View Controller (MVC) Architecture
- Components of MVC
- Setting Up a Spring MVC Application
- The Purpose of Dispatcher Servlets
- Spring Controllers
- Spring View Resolvers
- Adding Data using Spring Data Models
- Creating and Managing Forms in Spring
- Managing File Uploads
- Apache Tiles Integration
Spring: Aspect Oriented Programming (AOP)
In our module, you will learn how precisely Aspect Oriented Programming improves the weaknesses of Object Oriented Programming by segregating cross-cutting concerns.
- Limitations of Object Oriented Programming
- Introduction to Aspect-Oriented Programming
- Advantages of AOP
- Terminologies Associated with AOP
- Aspects
- โAdviceโ to Aspects
- Types of Advice โ Before, After, Around, Others
- Creating Annotation based Aspects
- Point Cut Expressions โ โWithinโ, โThisโ, โTargetโ
- Point Cut Designators
- AspectJ
Explore comprehensive insights into these subjects with our Full Stack Developer Courses in Bangalore.
Spring: Security
This module will help you configure authentications, set up robust Spring Security filters for authentication, and implement fine-grained authorizations.
- Spring: Security
- Securing Applications with Spring Security
- Spring Security Filters
- Configuring Authentications
- Spring Authorizations
- โRemember Meโ Functionality
Spring: Boot
In this module, you will see how Spring Boot helps in configuring the application through its auto-configuration and opinionated defaults.
- Introduction to Spring Boot
- Features of Spring Boot
- The Spring Boot Project Structure
- The Spring Boot Initializr
- Spring Boot Actuator
- Configuring the Spring Boot Server
- Spring Boot Application Properties
- Spring Vs Spring Boot
TypeScript Framework
In this chapter, you will learn how to build scalable applications with TypeScript. Master strong typing, interfaces, and decoratorsโthese key concepts for high readability and maintainability of code. Practical integration of popular frameworks, such as Angular and NestJS, will also be covered in detail to help you understand how TypeScript can help you really speed up both frontend and backend development. You will be empowered to be effective in writing efficient, type-safe applications so that they are fit for todayโs demanding software landscape. To learn more about Typescript, refer to the Full Stack Developer Tutorial.
TypeScript Framework
Youโll be exposed to the very basics of TypeScript within our TypeScript Framework moduleโlearn how to improve code reliability with its high-powered typing system.
- Introduction to TypeScript
- Advantages of TypeScript
- Overview of Node and Node Environment Setup
- Installing and Configuring TypeScript Engine
- Understanding Transpilation
Typescript Variables and Data Types
You will learn all the significant concepts of variable declaration and definition in this module of Variables and Data Types.
- Declaring and Defining Variables
- About โanyโ
- โvarโ Vs โletโ keywords
- Static and Dynamic Type
- Data types : String, Number, Array, Object, Tuple, Enum, Void and NULL
OOPS in TypeScript
You will learn the basics of OOPS (Object Oriented Programming) and how to define a class, create an object, and the syntax of TypeScript.
- Defining Class and Creating Objects
- Property, Methods and Constructors
- Handling Inheritance
- Types of Inheritance in TypeScript
- Understanding Access Modifiers
- Static Methods in TypeScript
- Understanding Interfaces in TypeScript
Programming with Angular โ 13
Youโll dive deep into state-of-the-art front-end development with Angular. Master the very latest features of Angular, including component architecture, TypeScript integration, and reactive programming using RxJS. Youโll be empowered to build these dynamic, responsive web apps on your own by using Angularโs robust CLI, which makes it easy to manage projects and deploy them. You will come out of this book knowing how to create easily and efficiently robust, modern experiences on the web. Angular forms a critical part of the Full Stack Developer Interview Questions and Answers and is a frequently asked about topic.
Introducing Angular โ 13
In this course, youโll be introduced to Angular-13, a powerful and versatile framework for building dynamic web applications. Youโll learn what Angular is, its advantages, and the differences between frameworks and libraries.
- What is Angular
- Advantages of using Angular
- Understanding Differences between a Framework and a Library
- Understanding Single Page Applications (SPA)
- SPA Vs. Traditional Applications
- Understanding the MVC Architecture
- MVC in Client and Server Side
- Elements of Angular
- Modules
- Data Binding Directives
- Templates
- Metadata
- Services
- Dependency Injection
- Angular Components
Angular โ Environment Setup
In this module, youโll learn to set up the Angular environment, including installing Node.js and understanding NPM, the benefits of using Angular CLI, creating an Angular app, exploring the application file structure, executing the app, performing code linting, and getting an introduction to Webpack.
- Installing Node.js and understanding Node Package Manager ( NPM )
- What is Angular CLI
- Advantages of using Angular CLI to manage Projects
- Installing Angular CLI
- Create a Angular App with CLI
- Angular Application File Structure
- Executing Angular Application
- Code Linting
- Installing and Understanding Webpack
Angular Modules
In this module, you will be learning about Angular Modules, including when and how to use them, the intricacies of NgModule, and the differences between Root, Feature, and Shared Modules. Youโll also master using providers, managing imports, and bootstrapping an Angular application.
- Understanding Angular Modules
- When to use Angular Modules
- Understanding NgModule
- Understanding Root Modules, Feature Modules, Shared Modules
- Using Providers, Managing Imports
- Bootstrapping Angular Application
Angular Components
Gain a deep understanding of Angular components, including their structure, interaction, and lifecycle.
- Understanding Components
- Understanding Components Structure
- Components Interaction and Component Lifecycle
- Using ngOnInt
- Exploring All Lifecycle Hooks
- Understanding Change Detection
- Working with Zone.js
- Working with Component Decorator and its Meta data
- Passing Data between Child and Parent
Angular โ Data and Event Bindings
In this module, you will look into Angularโs data and event bindings. You will master the basics of data binding and two-way data binding, style and class binding, event binding, and then also gain practical skills in element reference and event filtering.
- Understanding Data Binding
- Explaining Two-way Data Binding
- Working with Style Binding and Class Binding
- Understanding and Exploring Event Binding
- Element Reference and Event Filtering
Angular Directives and Custom Directives
In this module of the Java Full Stack Syllabus,You will learn Angular Directives, types of Directives, which include Structural and Attribute Directives, and how they can relax the functionality of your application within this module.
- What are Directives in Angular?
- Understanding Different types of Angular Directives
- Structural Directives Vs. Attribute Directives
- Exploring all the Angular Structural and Attribute Directives
- Creating Custom Attribute Directives
- Creating Custom Structural Directives
- Writing Attribute Directive Code
- Respond to User Initiated Events
- Pass and Receive property values
Gain a thorough understanding of the above concepts through our Full Stack Developer Course in Coimbatore.
Angular Pipes
In this module, youโll understand Angular Pipes, including their uses, parameterization, and how they differ from directives.
- Understanding Pipes in Angular
- Uses of Pipes
- Parameterizing Pipes
- Pipes Vs. Directives
- Exploring Pipes : Uppercase, Lowercase, Number, Percent, Currency, Date, Slice, Json
- Difference between Pure Pipes and Impure Pipes
- The PipeTransform Interface
- Chaining Pipes
- Creating Custom Pipes
Angular Services and Dependency Injection
In this module of the Java Full Stack Developer Course Syllabus, you will be looking into Angular Services and Dependency Injection, mastering how to create and utilize services for encapsulating business logic and sharing data across components.
- Understanding Services
- Purpose of using Services
- Creating Services in Angular
- Overview of Singleton Object
- Understanding Dependency Injection
- Injectors and Providers
- @Injectable() and Hierarchical DI
Angular Routing and Advanced Routing
This module will help you to master the Angular routing basicsโfrom configuring and defining routes to handling static and dynamic routes. Choose the Best Full Stack Developer Course Online if you want more knowledge on the topics.
- Understanding the Essentiality of Routing
- Configuring and Defining Routing in Angular Application
- Understanding the Routing Parameters
- Understanding Static and Dynamic Routes
- Passing and Fetching Route Parameters
- Creating Child Route
Angular Template Driven Forms
In this module of the Java Full Stack Developer Course Syllabus, you will learn how to set up and control forms with ngForm, access and manipulate form values, implement validations, work with Form Groups and Form Control classes, and use one-way data binding to enable seamless user interaction and data management.
- Introduction to Forms
- Overview of Template-Driven Forms
- Setting up Forms in Angular and Forms Control
- Understanding ngForm
- Accessing Form Values and setting default form values
- Understanding Various Forms States
- Form Validations
- Form Groups and Form Control Class
- Understanding One-Way Data Binding in Angular Forms
Angular Reactive Forms
You will learn about predefined Angular pipes, including Uppercase, Lowercase, Number, Percent, Currency, Date, Slice, and JSON. You will also master the difference between pure and impure pipes and their application during data transformation and presentation.
- Understanding Reactive Forms
- Template Driven Forms Vs. Reactive Forms
- Reactive Form Control and Sync View
- Dynamic Validations in Reactive Forms
- Getting Reactive Form Values
Angular Asynchronous Operations and Advanced HTTP
In this module of the Java Full Stack Developer Course Syllabus, Master Angular asynchronous operations, advanced HTTP handling, Event Emitters, custom events, ng-content, ng-container, and observables while effectively connecting to backend APIs.
- Understanding Event Emitter
- Transferring Data with Event Emitter
- Creating Custom Events and Trigger
- ng-content and ng-container
- Introduction to Async
- Understanding HTTP mechanism
- Handling HTTP Request and Response
- Introduction to Observable and Observer
- Call back Methods in Observable
- Creating Custom Observable
- Connecting backend and APIs
Angular Authorizations
Understand client-side and server-side authorization in Angular, learn to create and configure route guards, and follow advanced information on HTTP interceptors for the security of your apps.
- Understanding Client-side Authorization and Server Side Authorization
- Creating Guard in Angular
- Understanding Route Guard
- Setting up Route Guard
- Understanding HTTP Interceptors
Angular Animations
In this module of the Java Full Stack Syllabus, youโll learn Angular animations, starting with an introduction and setup and progressing through states and transitions, automatic property calculations, and animation timing.
- Introduction to Animations
- Animations Setup
- Understanding States & Transitions
- Entering and Leaving from States
- Animatable Properties
- Animatable Units
- Automatic Property Calculations
- Understanding Animation Timing
- Multistep Animation using Keyframes
Testing Angular Applications
In this module, you will get deep insights into testing Angular applications by first understanding what testing is and learning about unit testing.
- Introduction to Testing
- Unit Testing
- Setup Jasmine Framework
- Basics to Test Components
- Components Test Scenarios
- Introduction to Karma
- E2E Testing and Protractor
Angular Security and Internalization
In this module of the Java Full Stack Developer Course Syllabus, you will learn all the essential security practices for Angular applications, including how to sanitize dangerous values, use bypassSecurityTrust methods, CSRF protection, and internationalization with ng2-Translate.
- Importance of Security
- Security in Angular
- Sanitizing the Dangerous Value
- Trusting Values
- bypassSecurityTrustHtml
- bypassSecurity TrustScript
- bypassSecurityTrustStyle
- bypassSecurityTrustUrl
- bypassSecurityTrustResourceUrl
- Cross-site Request Forgery
- Pre-Compiled and runtime
- Using ng2-Translate
Angular Performance, Optimization and Deployment
You will learn different Angular performance optimization techniques, amongst which are those related to change detection strategies, leveraging web workers, and implementing lazy loading.
- Change Detection Strategy
- Understanding Web Workers
- Precompiling (AoT)
- Lazy Loading
- Deployment Best Practices
- Production Mode
Real-Time Project with Angular
You will work on a real-time Angular project that will give you hands-on experience in building dynamic and interactive web applications using it, as well as the core features associated with two-way data binding, components, services, and routing.