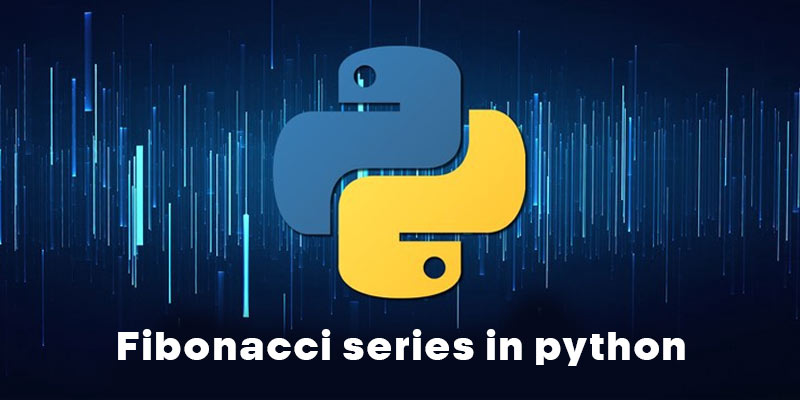
In this blog we will learn how to create a fibonacci sequence using python.But before that,
Fibonacci sequence follows a pattern where each number is the sum of the sum of the two preceding ones, starting from 0 and 1. That is,
Fibonacci sequence
Here are the first few fibonacci numbers of the sequence…
0, 1, 1, 2, 3, 5.……
The nth term in the sequence is the sum of (n-1)th and (n-2)th term.
Checkout this Online Python Course by FITA. FITA provides a complete Python course where you will be building real-time projects like Bitly and Twitter bundled with Career support.
Now let’s write programs using various methods to print the fibonacci terms until the input number.
Fibonacci Program In Python Using Recursions
def fib(num): if num <= 1: return num else: return (fib(num – 1) + fib(num – 2)) nu= int(input(“Enter the number of terms you want: “)) if nu <= 0: print(“Give a natural number”) else: for n in range(nu): print(fib(n),end=’,’) |
Output
Enter the number of terms you want: 4 0,1,1,2, |
Steps involved in the above program:
Fibonacci Program In Python With Dynamic Programming
FibTerms = [0, 1] def fib(n): if n <= len(FibTerms): return FibTerms[n – 1] else: term = fib(n – 1) + fib(n – 2) FibTerms.append(term) return term n=int(input(‘How many terms do you want’)) for i in range(n): print(fib(i),end=’,’) |
Output
Enter the number of terms you want: 9 1,0,1,1,2,3,5,8,13, |
Steps involved in the above code:
Fibonacci Program In Python Using While Loop
number = int(input(“How many terms? “)) n1, n2 = 0, 1 count = 0 if number <= 0: print(“Enter a positive integer”) elif number == 1: print(n1) else: while count < number: print(n1,end=’,’) sum= n1 + n2 n1,n2 = n2,sum count += 1 |
Steps involved in the above program
To get in-depth knowledge of Python along with its various applications and real-time projects, you can enroll in Python Training in Chennai or Python Training in Bangalore by FITA at an affordable price, which includes certification, support with career guidance assistance.