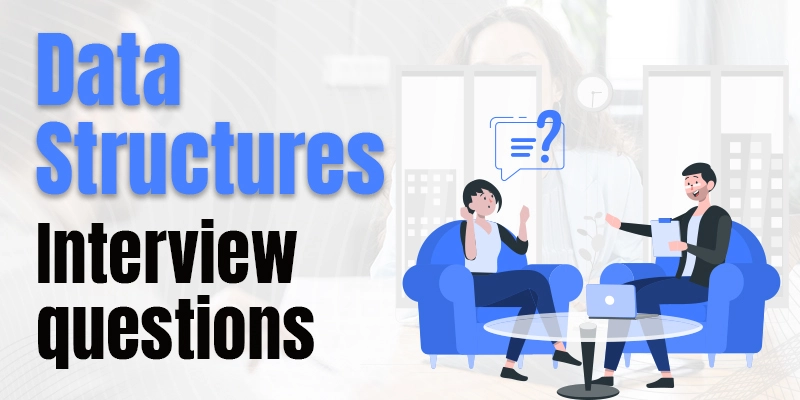
Navigating technical interviews can be a challenging experience, particularly when it involves mastering data structures and algorithms. With companies increasingly prioritising problem-solving skills, a solid grasp of DSA Interview Questions is crucial. Whether you’re a seasoned developer brushing up on your knowledge or a fresher preparing for your first interview, this comprehensive list of 50 essential topics will help you aware of DSA tipics during interviews. Also with Java Training in Chennai, you can master these algorithms below.
Data Structures and Algorithms
1. Array
An array is a group of elements stored in contiguous memory locations. All elements must be of the same data type, allowing for efficient access via indexing (e.g., `array[0]` for the first element). Arrays provide O(1) time complexity for access but O(n) for insertion and deletion unless at the end. The array data structure concept is one of the most commonly DSA interview questions. For freshers preparing for technical interviews, arrays often serve as a foundation for other data structures.
2. Linked Lists (Singly/Doubly)
- Singly Linked List: Each node contains data and a pointer to the next node. This structure allows for efficient insertions and deletions but requires O(n) time to access an element by index. Common DSA interview questions for freshers often focus on the advantages of linked lists over arrays.
- Doubly Linked List: Each node has pointers to the next and previous nodes, enabling traversal in both directions. This flexibility allows for more straightforward deletion and insertion, but it also requires additional memory. In DSA technical interview questions, interviewers may ask about the trade-offs of using singly versus doubly linked lists.
The implementation of Python List is similar to Vectors in C++ or ArrayList in JAVA. By taking the best Python Training in Chennai, you can learn important data structures for python.
3. Stack
A stack follows the Last-In-First-Out (LIFO) principle, where the last added element is removed first. It supports two primary operations:
- push (adding an element)
- pop (removing the top element)
Stacks are used in recursive function calls, expression evaluation, and backtracking algorithms. Familiarity with stacks can help candidates tackle various DSA questions for interviews, especially in coding tests.
4. Queue
A queue operates on a First-In-First-Out (FIFO) basis, with elements added at the rear and removed from the front. Basic operations include enqueue(adding) and dequeue (removing). Queues are essential for scheduling processes, breadth-first search, and task management. Understanding queues is vital for solving DSA interview questions and answers related to data processing.
5. Priority Queue
A priority queue extends the regular queue by associating a priority with each element. Elements are dequeued based on their priority rather than their order of arrival. Standard implementations use heaps. Priority queues are useful in algorithms like Dijkstra’s for shortest paths, frequently appearing in DSA basic interview questions that test knowledge of data structures.
6. Hash Table and Hashing
Sl No. | Hashing | Hash Table |
1 | Hashing is the process of transforming a key/string of char into another value. | The hash Table is a container to store the key-value pairs. |
2 | Hashing is a default method and can be defined by users also. | In Java, data structures like Hash Table, HashMap, and HashSet utilise hashing for data storage, while C++ employs similar concepts with structures such as map, multimap, unordered_map, and set. |
3 | Hashing is employed to produce a hash code of the Integer type. | We can choose any type of hash table depending on our requirements. |
4 | Hashing applies the same method for each key to produce hash codes. | Hashing utilises a consistent approach for every key to generate hash codes. |
Trees, lists, graphs, sorting algorithms, hashing tables and algorithms are used in machine learning. To Learn more about machine learning, consider the best Machine Learning Course in Chennai and start your career in AI.
7. Binary Search Tree (BST)
A BST is a binary tree in which each node can have up to two child nodes. For any given node, all values in the left subtree are less, and all in the right are greater. This structure allows for efficient searching, insertion, and deletion in average O(log n) time, though performance can degrade to O(n) in skewed trees. Understanding BSTs can be crucial for candidates facing DSA technical interview questions.
8. AVL Tree
An AVL tree is a self-balancing BST where the difference in heights between left and right subtrees (the balance factor) is at most one. This ensures that operations remain efficient (O(log n)) by performing rotations during insertion and deletion to maintain balance.
9. Red-Black Trees
A red-black tree is another self-balancing BST with specific properties, including node colour (red or black). These properties ensure balanced tree height and guarantee O(log n) time for search, insertion, and deletion. They are widely used in many programming languages’ standard libraries. Questions regarding red-black trees are often asked in DSA interview questions.
10. Graph Representation
Graphs can be presented in various ways:
- Adjacent Matrix: A 2D array where each cell (i, j) represents the presence of an edge between vertices i and j. This representation is efficient for dense graphs but consumes O(V^2) space. Graph representation is a common topic in data structures interview questions for freshers.
- Adjacent List: Each vertex has a list of its adjacent vertices. This is more space-efficient for sparse graphs, using O(V + E) space.
The demand for data-driven professionals is skyrocketing.
Learn Data Analytics at FITA Academy and fit yourself into the demand.
Explore Program11. Depth First Search (DFS)
It is a traversal algorithm that explores each branch as deeply as possible before backtracking. It can be implemented using recursion or a stack. It is used in pathfinding, topological sorting, and solving puzzles. Candidates should be prepared to discuss DFS in DSA coding questions and answers.
12. Breadth-First Search (BFS)
BFS explores a graph layer by layer, visiting all adjacent vertices before moving to the next level. It uses a queue for tracking nodes. BFS is essential in finding the shortest path in unweighted graphs and is also used in peer-to-peer networks and social networking applications. BFS-related questions are common in DSA technical interview questions.
13. Dijkstra’s Algorithm
This algorithm identifies the shortest path from the source vertex to all other vertices in a weighed graph with non-negative weights. It uses a priority queue to repeatedly select the nearest unvisited vertex and update the distances to its neighbours. Knowledge of Dijkstra’s algorithm is essential for tackling DSA interview questions for freshers.
14. Bellman-Ford Algorithm
The Bellman-Ford algorithm identifies the shortest paths from a single source but can handle graphs with negative edge weights. It relaxes all edges up to V-1 times (where V is the number of vertices), ensuring that the shortest path is found even with negative weights. Candidates might face this topic in DSA viva questions.
15. Floyd-Warshall Algorithm
This dynamic programming algorithm computes shortest paths between all pairs of vertices in a graph. It uses a 2D array to store distances and iteratively updates it to reflect the shortest paths. It can handle negative weights but not negative cycles.
16. Kruskal’s Algorithm
In Kruskalโs algorithm, all edges of the given graph are sorted in ascending order. The algorithm then adds edges and nodes to the Minimum Spanning Tree (MST) as long as the newly added edge does not create a cycle. It begins by selecting the edge with the smallest weight and concludes by including the edge with the largest weight.
17. Prim’s Algorithm
This is used to find the minimum spanning tree (MST) of a graph using a greedy approach. Starting from an arbitrary node, it adds the least expensive edge that connects a vertex in the MST to a vertex outside it, ensuring no cycles are formed. This algorithm is often covered in DSA interview questions.
18. Topological Sort Algorithm
This algorithm produces a linear ordering of vertices in a directed acyclic graph (DAG), ensuring that for every directed edge from vertex A to vertex B, vertex A precedes vertex B in the ordering. It can be performed using DFS or BFS. Candidates may encounter questions on topological sorting in DSA basic interview questions.
19. Union-Find Algorithm
The Union-Find algorithm manages disjoint sets and supports union and find operations efficiently. It uses techniques like path compression and union by rank to maintain efficiency, commonly used in network connectivity and Kruskal’s algorithm for MST. This algorithm is commonly asked in DSA technical interview questions.
20. Dynamic Programming (DP)
This is a technique for solving complex problems by breaking them down into simpler problems. By storing the results of these subproblems, DP avoids redundant calculations and improves efficiency, often solving problems in polynomial time. Mastery of DP is crucial for those preparing for DSA interview questions and answers.
Looking for a high-paying career? Data Science is thriving!
Enroll in our Data Science Course and master the skills to become a pro.
Explore Program21. Longest Common Subsequence (LCS)
This identifies the longest subsequence common to two sequences, which may not be contiguous. It uses a 2D DP table to build solutions incrementally, crucial in string matching and bioinformatics.
22. Longest Increasing Subsequence (LIS)
LIS finds the length of the longest subsequence in a given sequence of numbers that is strictly increasing. It can be solved using a DP approach or a combination of binary search and patience sorting for better efficiency.
23. 0/1 Knapsack Problem
In the 0/1 Knapsack problem, a set of items, each with a weight and value, must be selected to maximise total value without exceeding a given weight limit. This NP-complete problem can be approached using DP, where a table tracks maximum values for varying weights. Understanding knapsack problems is often crucial for DSA preparation for interviews.
24. Rod Cutting Problem
The rod-cutting problem involves cutting a rod into pieces to maximise revenue based on given piece prices. A DP approach iteratively calculates the maximum revenue for each length by considering all possible first cuts.
25. Matrix Chain Multiplication
This algorithm finds the most efficient way to multiply a sequence of matrices by determining the optimal order of multiplications and minimising the total number of scalar multiplications using a DP table. Questions regarding matrix operations frequently come up in DSA interview questions.
26. Fibonacci Sequence (DP Approach)
The Fibonacci sequence can be computed efficiently using DP by storing previously calculated values, reducing the exponential time complexity of naive recursion to O(n). This fundamental algorithm serves as a base for understanding more complex DP problems in DSA interview questions.
27. Greedy Algorithms
Greedy algorithms build solutions incrementally by choosing the best local option at each step. While they can provide optimal solutions for some problems (like activity selection), they may fail for others, requiring careful analysis. Candidates may face greedy strategy questions in DSA technical interview questions.
28. Huffman Coding
Huffman coding is a loss-less data compression technique that uses variable-length codes for encoding symbols based on their frequencies. It builds a binary tree and is widely used in data compression applications. Learn crucial algorithms and data compression techniques with a Data Science Course in Chennai.
29. Activity Selection Problem
This problem involves selecting the maximum number of mutually compatible activities from a set, given their start and finish times. A greedy approach efficiently solves it by prioritising the earliest finishing times. Questions related to this problem often appear in DSA interview questions for freshers.
30. Backtracking Algorithms
Backtracking is a recursive problem-solving technique that incrementally builds candidates for solutions and abandons those that fail to satisfy the problem’s constraints. It is commonly used in puzzles, permutations, and combinations.
AI is leading the way; Don’t get left behind
Master AI skills at FITA Academy and shape the future!
Explore Program31. N-Queens Problem
The N-Queens problem requires placing N queens on an N x N chessboard and hence no two queens threaten each other. It can be solved by backtracking and exploring all possible arrangements.
32. Sudoku Solver
Sudoku can be solved efficiently using a combination of backtracking and constraint programming. The algorithm fills cells while ensuring that Sudoku rules are maintained and backtracks whenever a conflict arises. Learning Sudoku-solving strategies will be helpful for those who are preparing for DSA interview questions and answers.
33. Subset Sum Problem
The subset sum problem determines whether a subset of a given set of integers can sum to a specified target. This NP-complete problem can be tackled using recursive or dynamic programming approaches.
34. Sliding Window Technique
This technique defines a moving range over input data (arrays or strings) to efficiently solve problems related to contiguous subarrays, allowing for O(n) time complexity in various scenarios. Familiarity with this method is beneficial for candidates facing DSA preparation for interview questions.
35. Two-Pointer Technique
The two-pointer technique uses two pointers to traverse data structures simultaneously, optimising performance in problems involving sorted arrays or linked lists. It is often applied in solving problems like triplets and pairs in sorted arrays. Candidates might encounter this technique in DSA technical interview questions.
36. Fast and Slow Pointer Technique
Also known as the Hare and Tortoise algorithm, this method uses two pointers moving at different speeds to detect cycles in linked lists. It efficiently determines whether a linked list has a cycle and is often asked in DSA interview questions for freshers.
37. Kadane’s Algorithm
Kadane’s algorithm finds the maximum sum of a contiguous subarray within a one-dimensional array in linear time. It iteratively updates a maximum sum and the current sum, often appearing in DSA coding questions and answers.
38. Merge Sort
Merge sort is a divide-and-conquer sorting algorithm that recursively divides an array into smaller subarrays, sorts them, and merges them back together. It operates in O(n log n) time, making it efficient for large datasets. Understanding merge sort is critical for DSA interview questions as they play a vital role in data analytics. If you are interested to learn all about data, then join a Data Analytics Course in Chennai.
39. Quick Sort
Quick sort is another divide-and-conquer sorting algorithm that selects a pivot element and partitions the array around it, recursively sorting the partitions. It has a mean time complexity of O(n log n) but can degrade to O(n^2) in the worst case.
40. Heap Sort
Heap sort is a comparison-based method using a binary heap to sort elements. It builds a max heap and then repeatedly extracts the maximum element, resulting in O(n log n) time complexity.
Boost Your Career with Cutting-Edge IT Courses through a Master Program at FITA Academy!
Enquire Now41. Radix Sort
Radix sort processes integers by their digits, sorting them based on digit significance. It’s particularly efficient for sorting large numbers of integers or fixed-size strings, operating in O(nk) time, where k is the number of digits.
42. Counting Sort
Counting sort is a non-comparison sorting algorithm that counts the occurrences of each distinct element and uses this count to determine the sorted positions. It is efficient for sorting integers in O(n + k) time, where k is the input value range.
43. Binary Search Algorithm
This search algorithm operates on sorted arrays by dividing the search interval in half. It efficiently locates a target element in O(log n) time, making it a fundamental algorithm often tested in DSA interview questions for freshers.
44. Exponential Search Algorithm
Exponential search identifies the target range in a sorted array, doubling the range until it exceeds the target, then applying binary search within that range for efficient searching.
45. Ternary Search
Ternary search divides the search interval into three parts instead of two, checking which third contains the target element. It’s less commonly used than binary search but can be effective in specific scenarios.
46. Tree Traversal Techniques
Tree traversal methods include:
In-order: Left, root, right (used for BSTs to retrieve sorted data).
Pre-order: Root, left, right (helpful for copying trees).
Post-order: Left, right, root (used for deleting trees).
Understanding tree traversal is crucial for solving various DSA interview questions and answers.
47. Floyd’s Cycle Detection Algorithm
This algorithm detects cycles in linked lists by using two pointers moving at different speeds. If they meet, a cycle exists; if not, the list is acyclic.
48. Bit Manipulation Techniques
Bit manipulation involves using bitwise operators to perform tasks efficiently. Techniques include checking bits, toggling bits, and shifting, which are crucial in competitive programming.
49. String Manipulation (KMP Algorithm, Rabin-Karp)
- KMP Algorithm: Uses preprocessing to create a partial match table, allowing for efficient substring searching in O(n + m) time. This algorithm is commonly tested in DSA interview questions.
- Rabin-Karp: Utilizes hashing to search for patterns, allowing for quick comparisons of substrings by calculating hash values.
50. Graph Coloring
Graph colouring involves assigning colours to vertices in a graph. This is to ensure no two adjacent vertices have the same colour. Graphic colouring is one of the frequently asked DSA technical interview questions.
Arming yourself with a robust understanding of data structures and algorithms is not just about landing a job; it’s about becoming a better programmer. By exploring these 50 interview topics, you can enhance your technical and problem-solving skills. Remember, the key to success in technical interviews depends on practice and understanding the principles. So, dive into these topics, refine your skills, and step into your next interview with confidence. Your dream job is just a code away!